Dungeon Listener
Hook on to the various dungeon events (like PreBuild, PostBuild events) using a custom script. Attach this script to the dungeon game object to automatically receive the notifications
Setup
Create a new C# script and inherit it from DungeonEventListener
like below
Next, drop this script on the dungeon game object. Whenever the dungeon get fully built, the OnPostDungeonBuild
function gets automatically called
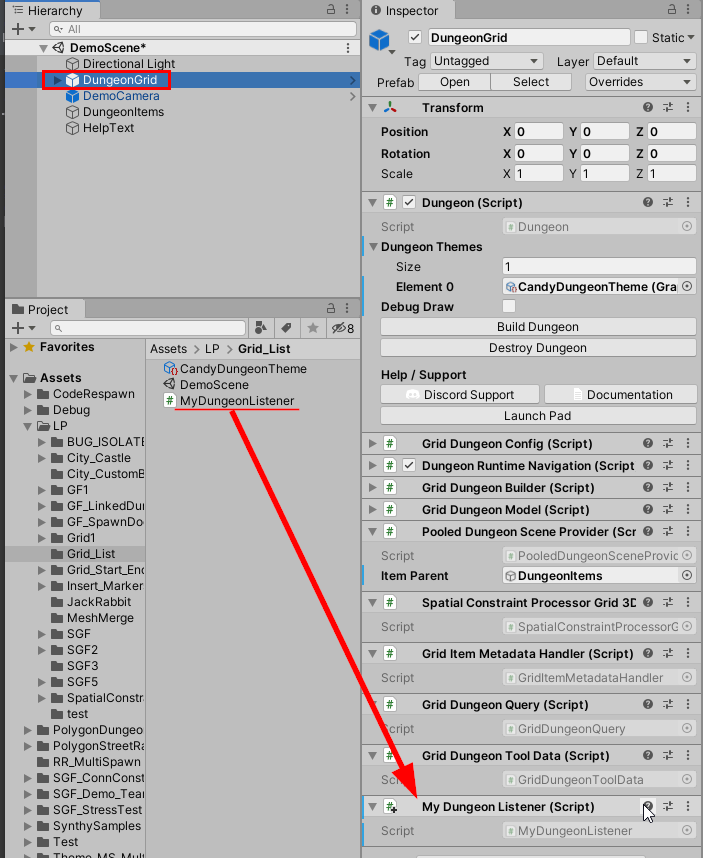
Get the full list of events from the API docs here
Event Functions
Here are a list of all the functions you can override to hook on to the various build events.
While building, the following events are called in this order:
Pre Build
(OnPreDungeonBuild)
- Called before the dungeon is builtPost Layout Build
(OnPostDungeonLayoutBuild)
- The layout is built in memory but nothing in spawned in the sceneMarkers Emitted
(OnDungeonMarkersEmitted)
- The layout markers are emitted in the scene for the theme engine to pick up. Nothing is spawned in the scene yet, it gives you an opportunity to modify the marker list before it is sent to the theme enginePost Build
(OnPostDungeonBuild)
- The dungeon is fully built
While destroying the dungeon, the following events are called in this order:
Pre Destroy
(OnPreDungeonDestroy)
- Called before the dungeon is destroyedDestroyed
(OnDungeonDestroyed)
- Called after the dungeon is destroyed
Pre Build
Called before the dungeon is about to be built
Post Layout Build
This is called after the dungeon layout has been built in memory, but before the prefabs have spawned. The dungeon would not have spawned anything at this stage, but the dungeon model would contain the layout data
Markers Emitted
The layout markers are emitted in the scene for the theme engine to pick up. Nothing is spawned in the scene yet, it gives you an opportunity to modify the marker list before it is sent to the theme engine
Post Build
Called after the dungeon is fully built
Pre Destroy
Called before the dungeon is destroyed
Destroyed
Called after the dungeon is destroyed